Collapsible Topics
Flow of Control Statements
Statements are the instructions given to the computer to perform any kind of action. Action may be in the form of data movement, decision making, etc. Statements form the smallest executable unit within a C++ program. Statements are always terminated by a semicolon.
Compound Statement
A compound statement is a grouping of statements in which each individual statement ends with a semi-colon. The group of statements is called a block. Compound statements are enclosed between a pair of braces ({}
). The opening brace ({
) signifies the beginning and the closing brace (}
) signifies the end of the block.
Null Statement
Writing only a semicolon indicates a null statement. Thus ;
is a null or empty statement. This is quite useful when the syntax of the language needs to specify a statement, but the logic of the program does not need any statement. This statement is generally used in for
and while
looping statements.
Conditional Statements
Sometimes the program needs to be executed depending upon a particular condition. C++ provides the following statements for implementing the selection control structure:
if
statementif-else
statement- Nested
if
statement switch
statement
If Statement
Syntax:
if (condition)
{
statement(s);
}
From the flowchart, it is clear that if the if
condition is true, the statement is executed; otherwise, it is skipped. The statement may either be a single or compound statement.
If-Else Statement
Syntax:
if (condition)
statement1;
else
statement2;
The given condition is evaluated first. If the condition is true, statement1
is executed. If the condition is false, statement2
is executed. Both statement1
and statement2
can be single or compound statements.
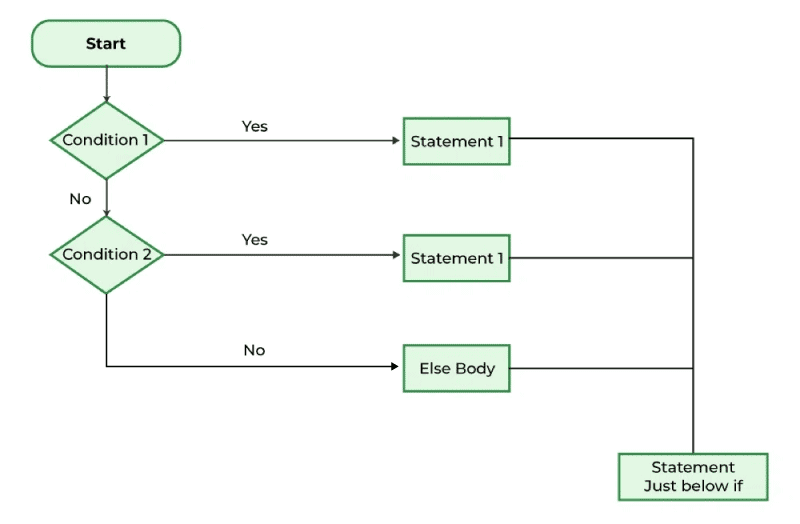
Examples:
if (x == 100)
cout << "x is 100";
if (x == 100)
cout << "x is 100";
else
cout << "x is not 100";
Nested If Statement
The if
block may be nested in another if
or else
block. This is called nesting of if
or else
blocks.
Syntax:
if (condition1)
{
if (condition2)
{
statement(s);
}
}
if (condition1)
statement1;
else if (condition2)
statement2;
else
statement3;
Example:
if (percentage >= 60)
cout << "1st division";
else if (percentage >= 50)
cout << "2nd division";
else if (percentage >= 40)
cout << "3rd division";
else
cout << "Fail";
Switch Statement
The if
and if-else
statements permit two-way branching, whereas the switch
statement permits multiple branching.
Syntax:
switch (var / expression)
{
case constant1: statement1;
break;
case constant2: statement2;
break;
...
default: statement3;
break;
}
The execution of the switch
statement begins with the evaluation of the expression. If the value of the expression matches a constant, the statements following that case execute sequentially until a break
is encountered. The break
statement transfers control to the end of the switch
statement. If no case matches, the default
case is executed.
Important Points:
- The expression in the
switch
statement must be of integer or character type. - The
default
case need not be the last case; it can be placed anywhere. - The case values do not need to be in any specific order.
Flow of Control: Looping Statements
Looping statements are also called Repetitive control structures. Sometimes, a set of statements needs to be executed multiple times, with one or more variables changing each time to produce different results. This type of program execution is called looping. C++ provides the following looping constructs:
while
loopdo-while
loopfor
loop
While Loop
Syntax:
while (condition)
{
statement(s);
}
The flow diagram indicates that a condition is first evaluated. If the condition is true, the loop body is executed, and the condition is re-evaluated. Hence, the loop body is executed repeatedly as long as the condition remains true. As soon as the condition becomes false, the program exits the loop and continues with the statement following the while
loop.
Do-While Loop
Syntax:
do
{
statements;
} while (condition);
Note: The loop body is always executed at least once. The key difference between the while
loop and the do-while
loop is the relative ordering of the conditional test and the loop body execution. In a while
loop, the loop repetition test is performed before each execution of the loop body; the loop body is not executed at all if the initial test fails. In a do-while
loop, the loop termination test is performed after each execution of the loop body, ensuring the loop body is executed at least once.
For Loop
For
loops are count-controlled loops, meaning the program knows in advance how many times the loop should be executed.
Syntax:
for (initialization; decision; increment/decrement)
{
statement(s);
}
The flow diagram indicates that three operations occur in a for
loop:
- Initialization: Initializes the loop control variable.
- Testing: Tests whether the loop control variable satisfies the condition (true or false).
- Update: Updates the loop control variable by incrementing or decrementing.
If the condition is true, the loop body executes, followed by an update of the loop control variable. The condition is checked again, and the process repeats. If the condition is false, the loop terminates.
Jump Statements
Jump statements unconditionally transfer program control within a function. C++ provides the following jump statements:
goto
statementbreak
statementcontinue
statementexit()
function
The goto
Statement
The goto
statement allows jumping to another point in the program.
goto pqr;
pqr: // pqr is a label
After executing the goto
statement, control transfers to the line after the label pqr
.
The break
Statement
The break
statement provides an immediate exit from a loop or a switch
structure. When executed in a loop, it immediately terminates the loop and transfers control to the statement following the loop.
The continue
Statement
The continue
statement is used in loops to skip the remaining part of the loop body and start a new iteration.
while (condition)
{
statement1;
if (condition)
continue;
statement2;
}
The continue
statement skips the rest of the loop body and begins the next iteration.
The exit()
Function
The execution of a program can be stopped at any point using the exit()
function, which optionally takes a status code to inform the calling program. The general format is:
exit(code);
The code
is an integer value, with 0
indicating successful execution. The value of the code may vary depending on the operating system.
Variable: Memory Concept
A variable is the name of the memory area where data is stored.
Programs shown in the previous section print text on the screen. This section introduces the concept of variables so our programs can perform calculations on data.
Program: Adding Two Numbers
Steps to solve this problem in C++:
- Step 1: Allocate memory for storing three numbers.
- Step 2: Store the first number in memory.
- Step 3: Store the second number in memory.
- Step 4: Add the two numbers and store the result in a third memory location.
- Step 5: Print the result.
Steps Explained
Step 1: Allocate memory for storing numbers. A memory location used to store data and given a symbolic name for reference is known as a variable. In this program, we need three variables:
int x;
int y;
int z;
Variables of the same type can also be declared in a single statement:
int x, y, z;
Step 2: Store a value in the first variable:
x = 25;
Step 3: Store a value in the second variable:
y = 10;
Step 4: Add the two numbers and store the result in the third variable:
z = x + y;
Step 5: Print the result:
cout << "The sum is " << z;
Complete Program
#include <iostream>
using namespace std;
int main()
{
// Declare variables of integer type
int x, y, z;
// Store values in variables
x = 25;
y = 10;
// Add numbers and store the result in z
z = x + y;
// Print the result
cout << "The sum is " << z;
return 0;
}
Output: The sum is 35
Identifiers
Symbolic names used for data items in a program are called identifiers. For example, x
, y
, and z
in the previous program are identifiers.
Rules for Identifiers
- An identifier can consist of alphabets, digits, and/or underscores.
- It must not start with a digit.
- C++ is case-sensitive, so uppercase and lowercase letters are treated differently.
- It should not be a reserved keyword.
Keywords
Reserved words in C++ with predefined meanings are called keywords. These cannot be used as identifiers. Some commonly used keywords are:
Keywords |
---|
asm |
auto |
bool |
break |
case |
catch |
char |
class |
const |
const_cast |
continue |
default |
delete |
do |
double |
dynamic_cast |
else |
enum |
explicit |
export |
extern |
false |
float |
for |
friend |
goto |
if |
inline |
int |
long |
mutable |
namespace |
new |
operator |
private |
protected |
public |
register |
reinterpret_cast |
return |
short |
signed |
sizeof |
static |
static_cast |
struct |
switch |
template |
this |
throw |
true |
try |
typedef |
typeid |
typename |
union |
unsigned |
using |
virtual |
void |
volatile |
wchar_t |
while |
Introduction
A programming language is a set of rules, symbols, and special words used to construct programs. Certain elements are common to all programming languages. Below, we discuss these elements in detail.
C++ Character Set
The character set is a set of valid characters that a language can recognize:
- Letters: A-Z, a-z
- Digits: 0-9
- Special Characters: Space, +, -, *, /, ^, \\, (), [], {}, =, !=, <>, ‘, “, $, ,, ;, :, %, !, &, ?, _, #, <=, >=, @
- Formatting Characters: Backspace, horizontal tab, vertical tab, form feed, and carriage return
Tokens
A token is a group of characters that logically belong together. Tokens in C++ include:
- Keywords
- Identifiers
- Literals
- Punctuators
- Operators
1. Keywords
These are reserved words in C++ with predefined meanings for the compiler. Refer to the previous section for details.
2. Identifiers
Symbolic names used for various data items in a program are called identifiers. Rules for forming identifiers:
- Can consist of alphabets, digits, and/or underscores
- Cannot start with a digit
- Are case-sensitive (uppercase and lowercase are distinct)
- Must not be a reserved word
3. Literals
Literals, often called constants, are data items that do not change during program execution. Types of literals:
- Integer Constants
- Character Constants
- Floating Constants
- String Literals
Integer Constants
Whole numbers without fractional parts. Types:
- Decimal: Sequence of digits, not starting with 0. Example: 124, -179, +108
- Octal: Sequence of digits starting with 0. Example: 014, 012
- Hexadecimal: Sequence preceded by
0x
or0X
. Example: 0x1A
Character Constants
Must contain one or more characters enclosed in single quotes. Example: 'A'
, '9'
. Escape sequences represent nongraphic characters (e.g., \n
for a new line).
Floating Constants
Numbers with fractional parts, written in fractional or exponent form. Example: 3.0, -17.0, -0.627
String Literals
A sequence of characters enclosed in double quotes. Automatically appended with \0
to denote the end. Example: "COMPUTER" is stored as "COMPUTER\0".
4. Punctuators
The following characters are used as punctuators in C++:
Punctuator | Purpose |
---|---|
[ ] | Indicate array subscript |
( ) | Indicate function calls, parameters, or grouping expressions |
{ } | Indicate start and end of compound statements |
, | Separator in function arguments |
; | Statement terminator |
: | Indicate labeled statements or conditional operator |
* | Pointer declaration or multiplication operator |
= | Assignment operator |
# | Preprocessor directive |
5. Operators
Special symbols for specific operations. Types of operators in C++:
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Unary Operators
- Assignment Operators
- Conditional Operators
- Comma Operators
Introduction
Operators are special symbols used for specific purposes. C++ provides many operators for manipulating data. There are six main types of operators:
- Arithmetical Operators
- Relational Operators
- Logical Operators
- Assignment Operators
- Conditional Operators
- Comma Operators
Arithmetical Operators
These operators are used to perform arithmetic operations:
Operator | Meaning |
---|---|
+ | Addition |
- | Subtraction |
* | Multiplication |
/ | Division |
% | Modulus |
The operators +, -, *, and / work with both integers and floating-point data types. The % operator is used only with integers.
Binary and Unary Operators
Binary Operators: Require two operands. Example: x + y
.
Unary Operators: Operate on a single variable. Example:
a = -50; a = +50;
Relational Operators
Relational operators test the relationship between two values. They return 0
if false and a non-zero value if true.
Relational Operators | Meaning |
---|---|
< | Less than |
<= | Less than or equal to |
== | Equal to |
> | Greater than |
>= | Greater than or equal to |
!= | Not equal to |
Logical Operators
Logical operators combine or negate relational expressions:
Operator | Meaning |
---|---|
|| | OR |
&& | AND |
! | NOT |
Assignment Operators
The =
operator assigns values. Compound assignment operators combine operations with assignment:
Operator | Example | Equivalent To |
---|---|---|
+= | A += 2 | A = A + 2 |
-= | A -= 2 | A = A - 2 |
*= | A *= 2 | A = A * 2 |
/= | A /= 2 | A = A / 2 |
%= | A %= 2 | A = A % 2 |
Increment and Decrement Operators
C++ provides ++
and --
to increment or decrement a value by 1:
- Pre-increment:
++variable
- Post-increment:
variable++
- Pre-decrement:
--variable
- Post-decrement:
variable--
Conditional Operator
The conditional operator (?:
) evaluates a condition:
big = (a > b) ? a : b;
If a > b
is true, big
gets a
. Otherwise, it gets b
.
Comma Operator
The comma operator evaluates expressions left to right. Only the last expression's value is considered.
sizeof Operator
The sizeof
operator determines the memory size of an object:
sizeof(char)
returns 1sizeof(float)
returns 4
Order of Precedence
The order of precedence determines the evaluation order of operators in an expression:
Order | Operators |
---|---|
First | () |
Second | *, /, % |
Third | +, - |
Basic Data Types
C++ supports a variety of data types, including:
Type | Description |
---|---|
int | Small integer number |
long int | Large integer number |
float | Small real number |
double | Double precision real number |
long double | Long double precision real number |
char | A single character |
The exact sizes and ranges of these types are system-dependent and can be found in the headers <climits>
and <cfloat>
.
C++ String Class
The string
class in C++ is used to handle sequences of characters. To use it, include the <string>
header file.
// Example program demonstrating the string class #include <iostream> #include <string> using namespace std; int main() { string mystring = "This is a string"; cout << mystring; return 0; }
Variable Initialization
A variable is a named location in memory that can store data. Variables must be declared before use.
float total; // Declaration int x, y; // Multiple variables in one declaration int a = 20; // Initialization with a value int b(30); // Constructor initialization
Constants
Constants are variables whose values cannot change during program execution. They are declared using the const
keyword.
const float PI = 3.1415; // Constant of type float const int RATE = 50; // Constant of type int const char CH = 'A'; // Constant of type char
Type Conversion
Type conversion is the process of converting one data type to another. There are two types:
1. Implicit Conversion
In implicit conversion, C++ automatically converts a lower data type to a higher data type in mixed expressions:
double a; int b = 5; float c = 8.5; a = b * c; // 'b' is converted to float, result converted to double
The order of data types is as follows (highest to lowest):
- long double
- double
- float
- long
- int
- char
2. Explicit Conversion
Explicit conversion (type casting) temporarily changes a variable's type:
totalPay = static_cast<double>(salary) + bonus; // 'salary' is cast to double before the addition
Introduction
The standard C++ library includes the header file <iostream>
, which facilitates input and output operations. These operations use the following stream objects:
cout
: Console outputcin
: Console input
Using cout
The cout
object is used to display output on the screen. It is combined with the insertion operator <<
.
cout << "Hello World"; // prints Hello World cout << 250; // prints 250 cout << sum; // prints the value of the variable 'sum'
Example of combining constants and variables in a single statement:
cout << "Area of rectangle is " << area << " square meter";
If area
is 24, the output will be:
Area of rectangle is 24 square meter
Using cin
The cin
object is used to take input from the user via the keyboard. It works with the extraction operator >>
to store the user input in variables.
int marks; cin >> marks; // Reads an integer value into 'marks'
Example program:
// Input/output example #include <iostream> using namespace std; int main() { int length, breadth, area; cout << "Please enter length of rectangle: "; cin >> length; cout << "Please enter breadth of rectangle: "; cin >> breadth; area = length * breadth; cout << "Area of rectangle is " << area; return 0; }
Output:
Please enter length of rectangle: 6 Please enter breadth of rectangle: 4 Area of rectangle is 24
Multiple inputs can be taken in a single statement:
cin >> length >> breadth; // Equivalent to: cin >> length; cin >> breadth;
Using cin
with Strings
The cin
object can be used to input strings. However, it stops reading at a space. For full-line input, use getline()
instead:
// cin and strings #include <iostream> #include <string> using namespace std; int main() { string name; cout << "Enter your name: "; getline(cin, name); cout << "Hello " << name << "!"; return 0; }
Output:
Enter your name: Mohsin Khan Hello Mohsin Khan!